Description
Use DNS Templates API to edit any specific DNS template.
DNS templates list
<?php
$user = 'user_name';
$pass = 'password';
$host = 'serverIP/hostname';
$url = 'https://'.rawurlencode($user).':'.rawurlencode($pass).'@'.$host.':2005/index.php?api=json&act=dns_template';
$post = array();
// Set the curl parameters
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
if(!empty($post)){
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($post));
}
// Get response from the server.
$resp = curl_exec($ch);
if(!empty(curl_error($ch))){
echo curl_error($ch); die();
}
// The response will hold a string as per the API response method.
$res = json_decode($resp, true);
echo "<pre>";
print_r($res['tmpl_list']);
echo "</pre>";
<?php
// Webuzo SDK file
include_once('/usr/local/webuzo/sdk/webuzo_sdk_v2.php');
$user = 'username';
$pass = 'password';
$host = 'serverIP/hostname;
// Create object of Webuzo_Admin_SDK class
$webuzo = new Webuzo_Admin_SDK($user, $pass, $host);
$res = $webuzo->read_dns_template();
// DNS Templates array
if(!empty($res)){
echo '<pre>';
print_r($res);
echo '</pre>';
}
?>
DNS Templates list is required to get the exact point to edit a specific templates record.
In the following parameters list the edit_record is the parameter where exact array key from list is needed to post to edit that record.
Please refer this following Output of DNS Templates list to know the edit_record value.
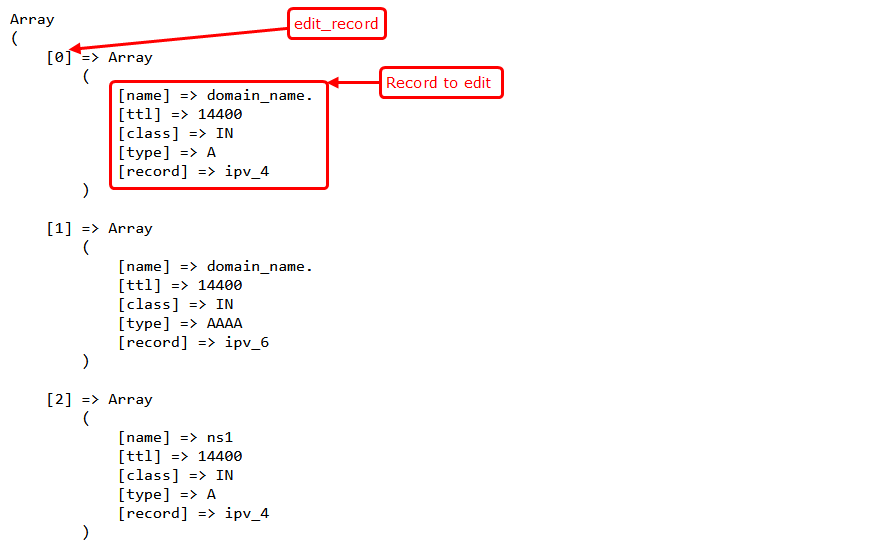
Sample code
Please refer following sample code for CURL, API and Webuzo SDK.
curl --insecure -d "edit_record=Array_key_to_edit" -d "name=prefix" -d "type=dns_type" -d "ttl=ttl_value" -d "record=address_or_ip" -u "user:password" -X POST "https://hostname_or_ip:2005/index.php?api=json&act=dns_template"
<?php
$user = 'user_name';
$pass = 'password';
$host = 'serverIP/hostname';
$url = 'https://'.rawurlencode($user).':'.rawurlencode($pass).'@'.$host.':2005/index.php?api=json&act=dns_template';
// Please refer the DNS templates list to post exact records to edit
$post = array('edit_record' => Array Key from DNS Templates list,
'type' => 'DNS_TYPE',
'name' => 'Prefix',
'ttl' => 14400,
'address' => 'Address/IP',
);
// Set the curl parameters
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
if(!empty($post)){
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($post));
}
// Get response from the server.
$resp = curl_exec($ch);
// Check if curl failed
if(!empty(curl_error($ch))){
echo curl_error($ch); die();
}
// The response will hold a string as per the API response method.
$res = json_decode($resp, true);
// Done ?
if(!empty($res['done'])){
print_r($res['done'][0]);
}else{
print_r($res['error'][0]);
}
<?php
// Webuzo SDK file
include_once('/usr/local/webuzo/sdk/webuzo_sdk_v2.php');
$user = 'username';
$pass = 'password';
$host = 'serverIP/hostname';
// Create object of Webuzo_Admin_SDK class
$webuzo = new Webuzo_Admin_SDK($user, $pass, $host);
$point = 'Array key from DNS templates list'
$name = 'name after edit';
$type = 'type after edit';
$ttl = 'ttl value after edit';
$address = 'Address/IP after edit';
$res = $webuzo->edit_dns_template($point, $name, $type, $ttl, $address);
// Done/Error
if(!empty($res['error'])){
print_r($res['error']);
}else{
print_r($res['done']['msg']);
}
?>
Output
DNS template edited successfully